Replacing a substring in a string [message #479987] |
Tue, 19 March 2013 02:32  |
iRANian
Messages: 4299 Registered: April 2011
Karma: 0
|
General (4 Stars) |
|
|
I need to be able to replace a substring in a string with my own string. For example:
"Herp derpEderpD"
I'd like to replace every occurrence of "derp" with "winter", so the final string looks like:
"Herp winterEwinterD"
How would I go about doing this? I see std::string allows me to do this, but I haven't figured out how to do this with C without doing tedious and dangerous pointer arithmetic and the C libraries shitty string functions. I also couldn't find a way to do this with StringClass, but I might have missed something.
Long time and well respected Renegade community member, programmer, modder and tester.
Scripts 4.0 private beta tester since May 2011.
My Renegade server plugins releases
|
|
|
Re: Replacing a substring in a string [message #479994 is a reply to message #479987] |
Tue, 19 March 2013 13:46   |
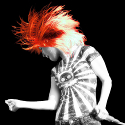 |
danpaul88
Messages: 5795 Registered: June 2004 Location: England
Karma: 0
|
General (5 Stars) |
|
|
There isn't a built-in method of doing this in C++ (never mind C), but it's not that hard. 5 seconds on Google gives a workable example;
http://stackoverflow.com/questions/1452501/string-replace-in-c
A more efficient solution would be to count how many instances of <search> there are, allocate a new buffer with the required memory size (1 + originalLength + (( length(replace)-(length(match) ) * nMatches)) and then memcpy the unmodified bits of string and as many instances of <replace> as required to build the new string.
Avoids doing unnecessary and costly memory allocations that would be involved in doing it iteratively as in the above example.
[Updated on: Tue, 19 March 2013 13:53] Report message to a moderator
|
|
|
Re: Replacing a substring in a string [message #479995 is a reply to message #479987] |
Tue, 19 March 2013 13:54   |
iRANian
Messages: 4299 Registered: April 2011
Karma: 0
|
General (4 Stars) |
|
|
iRANian wrote on Tue, 19 March 2013 02:32 | How would I go about doing this? I see std::string allows me to do this, but I haven't figured out how to do this with C without doing tedious and dangerous pointer arithmetic and the C libraries shitty string functions. I also couldn't find a way to do this with StringClass, but I might have missed something.
|
Long time and well respected Renegade community member, programmer, modder and tester.
Scripts 4.0 private beta tester since May 2011.
My Renegade server plugins releases
|
|
|
|
Re: Replacing a substring in a string [message #479997 is a reply to message #479987] |
Wed, 20 March 2013 00:56   |
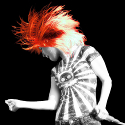 |
danpaul88
Messages: 5795 Registered: June 2004 Location: England
Karma: 0
|
General (5 Stars) |
|
|
std::string::replace replaces a set of characters between indexes N and M with a different set of characters. Whilst it can be used as a substring replacement it takes a bit of finangling to make it work and it still needs an iterative approach so it will potentially make lots of unnecessary intermediate buffer reallocations if the new string is >= the length of the string being replaced.
[Updated on: Wed, 20 March 2013 00:56] Report message to a moderator
|
|
|
Re: Replacing a substring in a string [message #479998 is a reply to message #479987] |
Wed, 20 March 2013 01:24   |
iRANian
Messages: 4299 Registered: April 2011
Karma: 0
|
General (4 Stars) |
|
|
Yeah that's what I'm afraid about.
@Blacky: I mean the standard C library. For some reason there's no version of strtok() that takes a substring as delimiter, just a char array of delimiters which are evaluated one by one.
Long time and well respected Renegade community member, programmer, modder and tester.
Scripts 4.0 private beta tester since May 2011.
My Renegade server plugins releases
|
|
|
|
|
Re: Replacing a substring in a string [message #480010 is a reply to message #479987] |
Thu, 21 March 2013 06:14   |
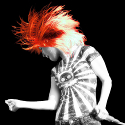 |
danpaul88
Messages: 5795 Registered: June 2004 Location: England
Karma: 0
|
General (5 Stars) |
|
|
Depends on a few things. If you just want pointers to both strings and don't mind them staying in the same memory block as each other you can write over the first character of the strstr result with a null character and advance its position by <length of search string> to get the pointer to the second string, using the original pointer as the pointer to the first string. Repeat until there are no more matches as necessary, either acting on each bit of string one at a time or accumulating the pointers in a vector or other storage container (pre-allocate it to a decent size first to avoid reallocations mid-processing as much as possible).
That's just one way of doing it off the top of my head. It's destructive to the source string (you could copy it first if this is a problem) but it allows you to split a string into multiple substrings. This is equivilent to how strtok works.
[Updated on: Thu, 21 March 2013 06:15] Report message to a moderator
|
|
|
Re: Replacing a substring in a string [message #480013 is a reply to message #479987] |
Thu, 21 March 2013 10:29  |
iRANian
Messages: 4299 Registered: April 2011
Karma: 0
|
General (4 Stars) |
|
|
I think I could do it with the StringClass truncate left and right functions after obtaining the position of the start of the matched substring.
I just need to know now how to convert the pointer returned by strstr() into a position into the base string, I assume it should be simple pointer arithmetic?
Long time and well respected Renegade community member, programmer, modder and tester.
Scripts 4.0 private beta tester since May 2011.
My Renegade server plugins releases
|
|
|